What is Django?
Python-based web framework used for rapid development of web applications.
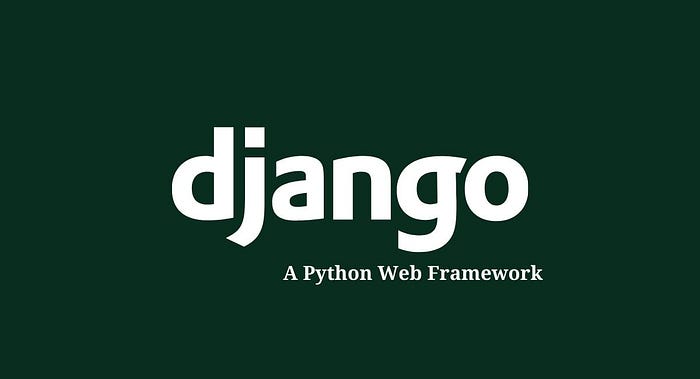
Installing Django + Setup
pip install django
Copy
Creating a project
The below command creates a new project named projectName
django-admin startproject projectName
Copy
Starting a server
The below command starts the development server.
python manage.py runserver
Copy
Django MVT
Django follows MVT(Model, View, Template) architecture.
Sample Django Model
The model represents the schema of the database.
from django.db import models
class Product(models.Model): # Product is the name of our model
product_id=models.AutoField
Copy
Sample views.py
View decides what data gets delivered to the template.
from django.http import HttpResponse
def index(request):
return HttpResponse("Django CodeWithHarry Cheatsheet")
Copy
Sample HTML Template
A sample .html file that contains HTML, CSS and Javascript.
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<meta http-equiv="X-UA-Compatible" content="ie=edge">
<title>CodeWithHarry Cheatsheet</title>
</head>
<body>
<h1>This is a sample template file.</h1>
</body>
</html>
Copy
Views in Django
Sample Function-Based Views
A python function that takes a web request and returns a web response.
from django.http import HttpResponse
def index(request):
return HttpResponse("This is a function based view.")
Copy
Sample Class-Based Views
Django’s class-based views provide an object-oriented way of organizing your view code.
from django.views import View
class SimpleClassBasedView(View):
def get(self, request):
pass # Code to process a GET request
Copy
URLs in Django
Set of URL patterns to be matched against the requested URL.
Sample urls.py file1
from django.contrib import admin
from django.urls import path
from . import views
urlPatterns = [
path('admin/', admin.site.urls),
path('', views.index, name='index'),
path('about/', views.about, name='about'),
]
Copy
Sample urls.py file2
from django.urls import include, path
urlpatterns = [
# ... snip ...
path('community/', include('aggregator.urls')),
path('contact/', include('contact.urls')),
# ... snip ...
]
Copy
Forms in Django
Similar to HTML forms but are created by Django using the form field.
Sample Django form
What is Django?
Python-based web framework used for rapid development of web applications.
Installing Django + Setup
pip install django
Copy
Creating a project
The below command creates a new project named projectName
django-admin startproject projectName
Copy
Starting a server
The below command starts the development server.
python manage.py runserver
Copy
Django MVT
Django follows MVT(Model, View, Template) architecture.
Sample Django Model
The model represents the schema of the database.
from django.db import models
class Product(models.Model): # Product is the name of our model
product_id=models.AutoField
Copy
Sample views.py
View decides what data gets delivered to the template.
from django.http import HttpResponse
def index(request):
return HttpResponse("Django CodeWithHarry Cheatsheet")
Copy
Sample HTML Template
A sample .html file that contains HTML, CSS and Javascript.
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<meta http-equiv="X-UA-Compatible" content="ie=edge">
<title>CodeWithHarry Cheatsheet</title>
</head>
<body>
<h1>This is a sample template file.</h1>
</body>
</html>
Copy
Views in Django
Sample Function-Based Views
A python function that takes a web request and returns a web response.
from django.http import HttpResponse
def index(request):
return HttpResponse("This is a function based view.")
Copy
Sample Class-Based Views
Django’s class-based views provide an object-oriented way of organizing your view code.
from django.views import View
class SimpleClassBasedView(View):
def get(self, request):
pass # Code to process a GET request
Copy
URLs in Django
Set of URL patterns to be matched against the requested URL.
Sample urls.py file1
from django.contrib import admin
from django.urls import path
from . import views
urlPatterns = [
path('admin/', admin.site.urls),
path('', views.index, name='index'),
path('about/', views.about, name='about'),
]
Sample urls.py file2
from django.urls import include, path
urlpatterns = [
# ... snip ...
path('community/', include('aggregator.urls')),
path('contact/', include('contact.urls')),
# ... snip ...
]
Forms in Django
Similar to HTML forms but are created by Django using the form field.
Sample Django form
from django import forms
# creating a form
class SampleForm(forms.Form):
name = forms.CharField()
description = forms.CharField()